Can a web page truly feel dynamic and responsive without the jarring interruption of a full reload? The answer, emphatically, is yes thanks to the magic of Asynchronous JavaScript and XML, better known as AJAX.
AJAX, a pivotal web development technique, empowers web pages to communicate with a web server behind the scenes. This communication happens asynchronously, meaning the user isn't forced to wait while the entire page refreshes. Instead, only the necessary parts of the page are updated, leading to a smoother, more interactive user experience. This is a cornerstone of modern web applications, underpinning the dynamic nature we've come to expect.
AJAX isn't a programming language itself; rather, it's a set of web development techniques. It brings together a collection of existing technologies. Its core components include:
- Fixer To Fabulous Net Worth Exploring The Success Of Hgtvs Beloved Duo
- Net Worth Of Brian Kilmeade A Comprehensive Look At His Finances And Success
- HTML and CSS: For structuring and styling the user interface.
- JavaScript: The engine that makes AJAX work, enabling interaction with the server and manipulating the web page content.
- XML (or JSON): Used for formatting the data received from the server. JSON (JavaScript Object Notation) is now more commonly used because it's lighter and easier to parse.
- XMLHttpRequest (XHR) Object: This is the backbone of AJAX, enabling the browser to communicate with the server in the background.
The process works like this: When a user interacts with a web page (e.g., clicks a button, submits a form), JavaScript, using the XMLHttpRequest object, sends a request to the web server. The server processes the request and sends back data, usually in XML or JSON format. JavaScript then uses this data to update specific parts of the web page without a full reload.
Aspect | Details |
---|---|
Core Function | Enables web pages to update content dynamically without full reloads. |
Underlying Technologies | HTML, CSS, JavaScript, XML (or JSON), and the XMLHttpRequest object. |
Communication Style | Asynchronous: Requests are sent to the server in the background, without blocking the user's interaction with the page. |
Primary Benefit | Enhanced user experience through increased responsiveness and interactivity. |
Data Format | Typically uses JSON (JavaScript Object Notation) for efficient data transfer. XML can also be used. |
Common Usage Cases | Dynamic content loading, form submission without page refresh, real-time updates, and creating single-page applications (SPAs). |
Advantages | Improved user experience, reduced server load (in some cases), faster loading times, and more dynamic web interactions. |
Disadvantages | Can increase complexity, requires proper error handling, and can pose security challenges if not implemented carefully (e.g., cross-site scripting). |
Alternatives | WebSockets, Server-Sent Events (SSE), and frameworks such as React, Angular, and Vue.js. |
One of the most significant advantages of AJAX is the improved user experience. Users can interact with a web page without the frustrating wait times associated with full page reloads. This leads to a more fluid and intuitive experience, keeping users engaged and enhancing overall satisfaction.
AJAX also contributes to faster loading times. By only updating specific parts of the page, less data needs to be transferred, leading to quicker response times. This is especially beneficial for users with slower internet connections.
- Mike Smith Net Worth A Comprehensive Insight Into His Wealth And Career
- Brett Berish Net Worth Unveiling The Success Behind The Luc Belaire Ceo
AJAX opens up a world of possibilities for creating more dynamic web interactions. Features like auto-suggestions in search bars, instant validation of form inputs, and real-time chat applications become easily achievable with AJAX. This ability to update content in real time makes web applications more engaging and useful.
To use AJAX, developers can leverage the XMLHttpRequest object directly or, more commonly, use libraries like jQuery, which simplifies the process significantly. JQuery's `.ajax()` method provides a streamlined way to send and receive data. Here's a basic example:
$.ajax({url: "your_server_script.php", // The URL of the server-side scripttype: "POST", // The HTTP method (GET, POST, etc.)data: { key: "value" }, // Data to send to the serversuccess: function(response) { // Function to execute on successful response// Handle the response from the server$("#result").html(response); // Update the HTML element with id "result"},error: function(xhr, status, error) { // Function to execute on error// Handle any errorsconsole.error(error);}});
This code sends a POST request to "your_server_script.php". It sends some data and, upon success, updates the content of an HTML element with the ID "result." Error handling is also included.
The parameters and options you can use with the `$.ajax()` method are quite extensive. Here are some important ones:
- `url` (String, Required): The URL of the server-side resource to which the request is sent.
- `type` (String, Default: "GET"): The HTTP method to use for the request (e.g., "GET", "POST", "PUT", "DELETE").
- `data` (Object or String): Data to be sent to the server. Can be an object (which jQuery will automatically encode) or a string.
- `dataType` (String, Default: Intelligent Guess (xml, json, script, or html)): The type of data expected back from the server. This helps jQuery parse the response correctly (e.g., "json", "xml", "html", "text").
- `success` (Function): A function to be called if the request succeeds. It receives the response data, the status of the request, and the XMLHttpRequest object as arguments.
- `error` (Function): A function to be called if the request fails. It receives the XMLHttpRequest object, a string describing the type of error, and an exception object (if applicable) as arguments.
- `beforeSend` (Function): A function to be called before the request is sent. Allows you to modify the XMLHttpRequest object.
- `complete` (Function): A function to be called when the request finishes (after success and error).
- `timeout` (Number): Sets a time limit (in milliseconds) for the request. If the request takes longer than the timeout, the request will be aborted.
- `async` (Boolean, Default: true): Whether to perform the request asynchronously (true) or synchronously (false). Synchronous requests can block the browser, so asynchronous requests are generally preferred.
- `cache` (Boolean, Default: true for GET requests, false otherwise): Whether to cache the request. Setting this to `false` is often useful to prevent the browser from caching the results of GET requests.
- `contentType` (String, Default: "application/x-www-form-urlencoded; charset=UTF-8"): The MIME type of the content being sent to the server.
- `processData` (Boolean, Default: true): Whether to process the data to be sent to the server. If set to `false`, data will be sent as is.
Let's consider a more practical example using the `fetch()` API, which is a modern and often simpler approach than using the `XMLHttpRequest` object directly.
fetch('your_server_endpoint.php', { method: 'POST', // or 'PUT', 'DELETE', etc. headers: {'Content-Type': 'application/json' // Tell the server we're sending JSON }, body: JSON.stringify({ // Convert the data to JSONkey1: 'value1',key2: 'value2' })}).then(response => response.json()) // Parse the JSON response.then(data => { // Do something with the data console.log('Success:', data); document.getElementById('result').innerHTML = data.message;}).catch((error) => { console.error('Error:', error);});
This example uses `fetch()` to send a POST request to `your_server_endpoint.php`. The request includes a JSON payload in the body. The `.then()` methods handle the response, parsing it as JSON and then doing something with the returned data. Error handling is managed by the `.catch()` block.
The advantages of using `fetch()` include a cleaner and more concise syntax compared to `XMLHttpRequest`. It also uses Promises, making it easier to handle asynchronous operations. However, it's important to note that `fetch()` has limited browser support compared to using jQuery's `.ajax()` which might be a factor in some projects.
AJAX is not without its potential pitfalls. Security is a major consideration. Because AJAX requests are made from the client-side (the user's browser), they are susceptible to Cross-Site Scripting (XSS) attacks. This involves malicious scripts being injected into the web page to steal user data or perform actions on behalf of the user. Careful input validation on the server-side is crucial to mitigate this risk. Developers must sanitize all data received from users before using it. Furthermore, AJAX requests can sometimes be exploited through Cross-Site Request Forgery (CSRF) attacks.
Another potential challenge is that AJAX can increase the complexity of web applications. Debugging can be more difficult because issues might not be immediately apparent. Using browser developer tools and debugging techniques are therefore very important.
Also, managing the state of an AJAX-driven application can be tricky. Asynchronous nature can cause problems with the flow of application. Handling complex data transformations and coordinating multiple AJAX requests also can become more complicated.
Considerations related to SEO: Search engines may have difficulty indexing content that is loaded dynamically via AJAX. Its essential to make sure important content is also available in a way that search engine crawlers can access it. This often involves server-side rendering or using techniques that allows search engines to crawl and index the application. Alternatively, developers may utilize Server-Side Rendering (SSR) with frameworks like React, Angular, or Vue.js, or employ techniques like pre-rendering to ensure search engines can effectively crawl and index the content.
Ajax provides numerous benefits, including the ability to update web pages without requiring a full reload of the entire page. This leads to a more responsive user experience, reduced bandwidth usage, and faster loading times, enhancing overall user engagement. AJAX's asynchronous nature enables web pages to communicate with servers in the background, allowing updates to specific portions of a page without interrupting the user's activities.
However, AJAX presents several challenges, including increased complexity, security concerns (such as cross-site scripting attacks), and SEO considerations. Developers must carefully implement input validation, implement CSRF protection, and make content accessible to search engine crawlers to address these potential pitfalls. The management of asynchronous requests, state, and error handling adds to the complexity of AJAX-based applications.
Many modern web frameworks and libraries, such as React, Angular, and Vue.js, have built-in mechanisms for handling AJAX requests and managing the complexities of dynamic content updates, and they also offer robust features for client-side rendering and state management.
Long polling, is a real-time communication technique that is often implemented within the framework of AJAX. It involves the client sending a request to the server, and the server holds the connection open until new data is available or a timeout occurs. When new data is available, the server sends the data to the client and closes the connection. The client then immediately initiates a new request, and the cycle repeats. This approach simulates a real-time connection, which can be useful for applications like chat applications and live dashboards. However, long polling is less efficient than WebSockets because it involves more overhead from HTTP requests, making it less suitable for applications that demand frequent, real-time updates.
In the context of AJAX implementations, error handling is essential. The `error` parameter in jQuery's `.ajax()` method and the `catch` block in `fetch()` are fundamental. These functions enable developers to gracefully handle situations where requests might fail, such as network errors or server issues. Proper error handling is crucial to prevent user frustration and ensure the application behaves predictably. The error handlers provide valuable debugging information and prevent the application from crashing.
WebSockets are an alternative to AJAX, providing a persistent connection between the client and the server. WebSockets are especially efficient for real-time applications. Unlike AJAX, WebSockets establish a persistent, bidirectional communication channel. The server can push updates to the client without the client having to request them. This reduces overhead and latency, making WebSockets suitable for applications where real-time data exchange is important, such as online games, collaborative editing tools, and live data feeds. However, WebSockets do require more server resources and are not always supported by all environments.
Server-Sent Events (SSE) represent another technology alternative. With SSE, the server can "push" data updates to the client over a single HTTP connection. SSE is similar to WebSockets in that it enables the server to send updates to the client automatically. However, SSE is unidirectional, meaning that the client can only receive updates from the server, not send data back in the same way. SSE is simpler to implement than WebSockets, and it's better suited for applications that need to receive a stream of updates from the server, such as live news feeds and stock tickers. However, for applications requiring bi-directional communication, WebSockets is the preferred choice.
Frameworks like React, Angular, and Vue.js simplify and streamline AJAX usage. These frameworks provide powerful tools and abstractions for creating complex web applications. They offer ways to handle HTTP requests, update the user interface in response to data changes, and manage the application's state efficiently. They also offer templating systems, virtual DOM updates, and component-based architectures, enabling developers to build modular, maintainable, and scalable applications. Utilizing these frameworks also often aids in more robust error handling and in handling the intricacies of asynchronous operations.
When choosing a framework, developers should consider project requirements, team familiarity, and the performance needs of the application. React is often selected for its flexibility and its virtual DOM, which allows efficient updates to the user interface. Angular is popular for large, complex applications due to its structure and extensive features. Vue.js is praised for its ease of use and versatility, making it a good choice for various types of projects.
Beyond the initial learning curve, incorporating AJAX effectively is vital. Here are some core best practices.
- Plan your requests: Before writing any code, design what data your application needs and how that data will be structured. This will save time in the long run.
- Handle errors gracefully: Always include robust error handling to identify and respond to possible network or server failures.
- Use JSON or a similarly efficient format: Keep in mind how much data transfer is taking place; selecting a lightweight format will keep applications faster.
- Consider caching: For requests that return data that doesnt frequently change, caching will save time.
- Thorough Testing: Thorough testing of all AJAX features, across various browsers, is crucial to ensure proper functionality.
AJAX is a cornerstone of modern web development, a technology that empowers web pages to provide dynamic, responsive, and engaging experiences. By mastering its core concepts and leveraging its benefits, developers can create applications that are not only faster and more efficient, but also provide a better user experience.
The impact of AJAX is far-reaching. From interactive web applications to sophisticated single-page applications (SPAs), AJAX is essential to a modern web experience. AJAX has evolved into a widely adopted standard, reshaping the landscape of web design. By understanding its capabilities and limitations, developers can create web applications that are both engaging and efficient.
The future of AJAX is bright, particularly with modern frameworks that simplify implementation, increasing performance, and enhancing user interactions. While it does have some drawbacks, it remains a pivotal technology that drives the evolution of the internet.
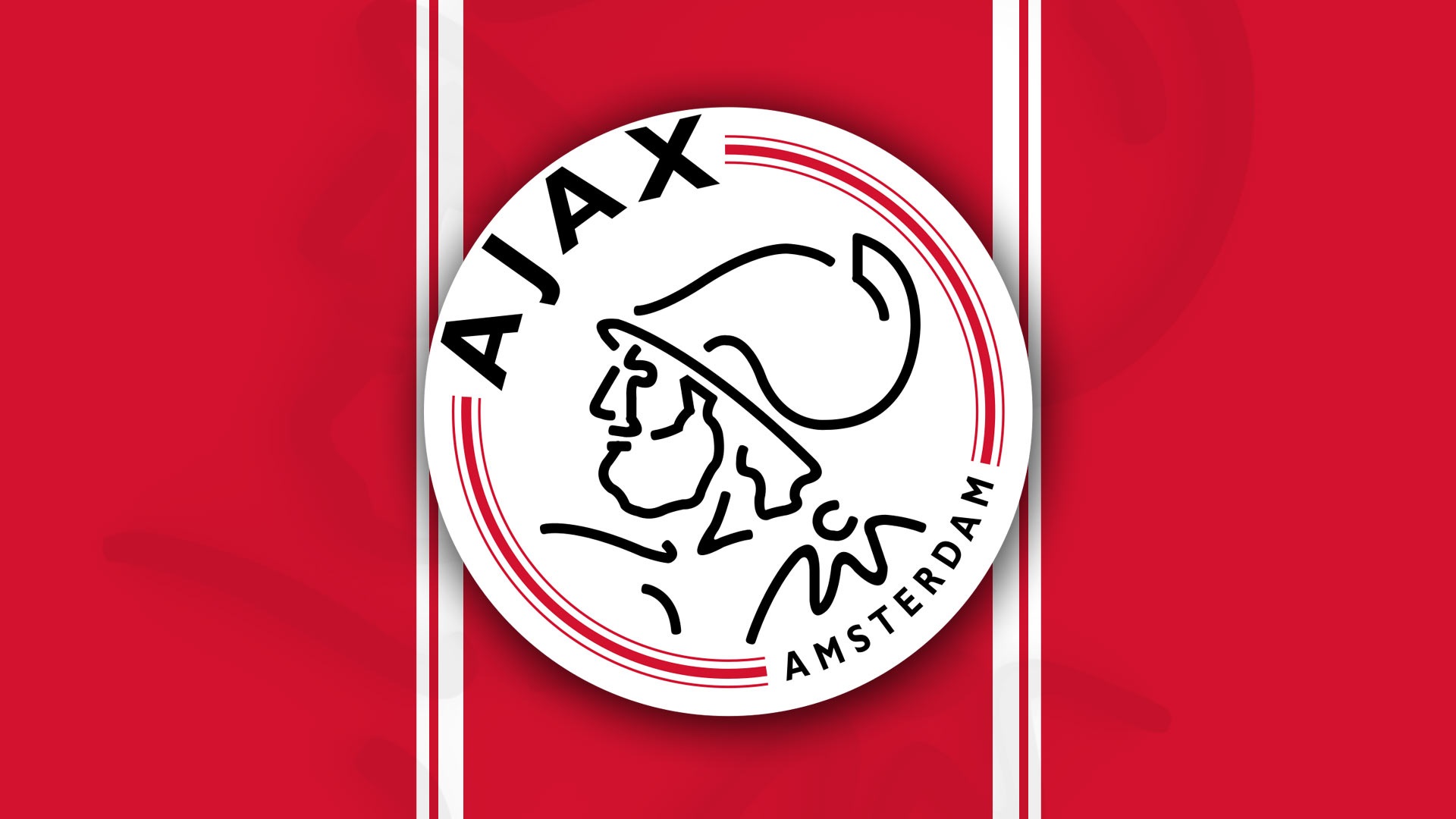
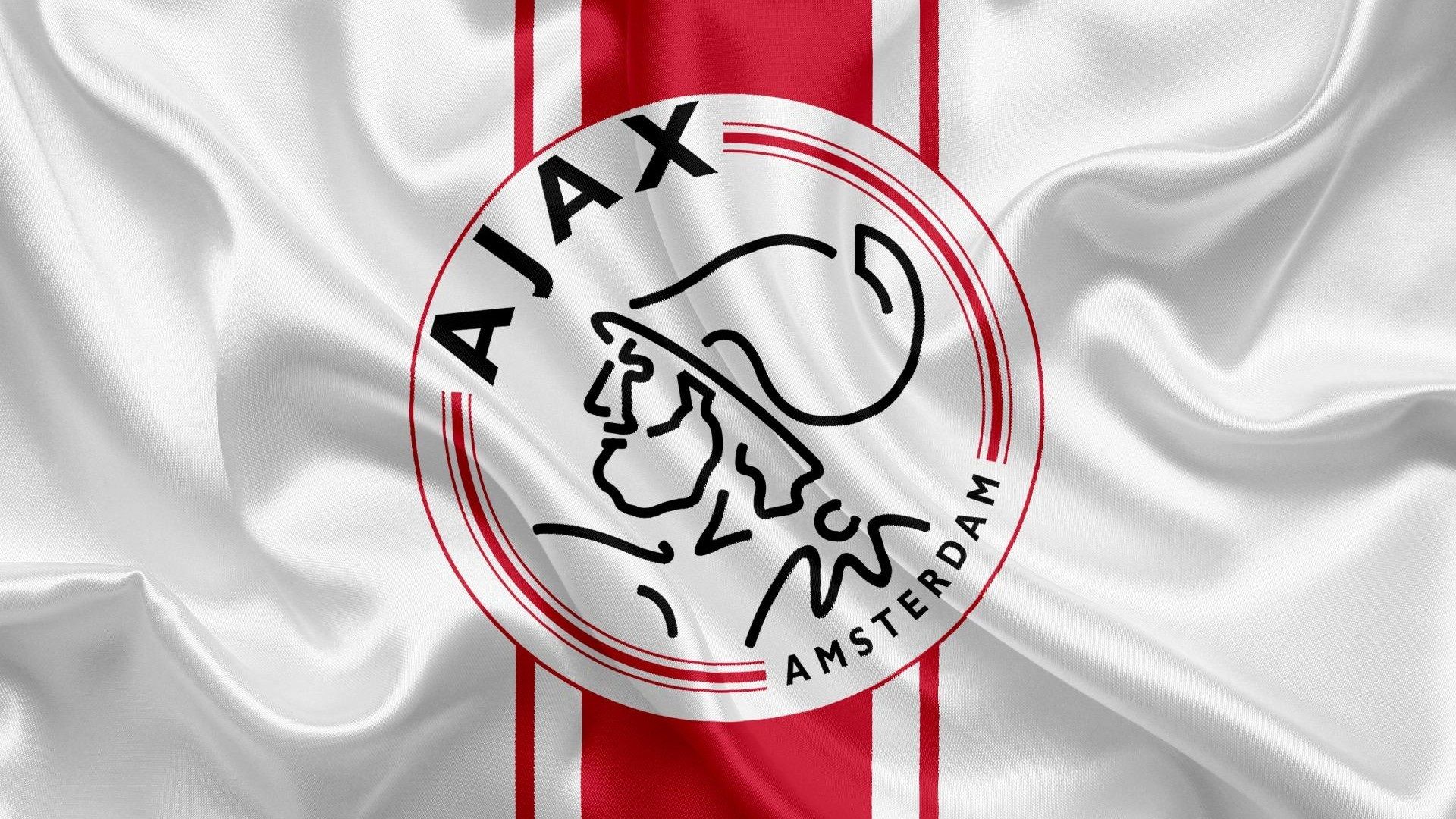
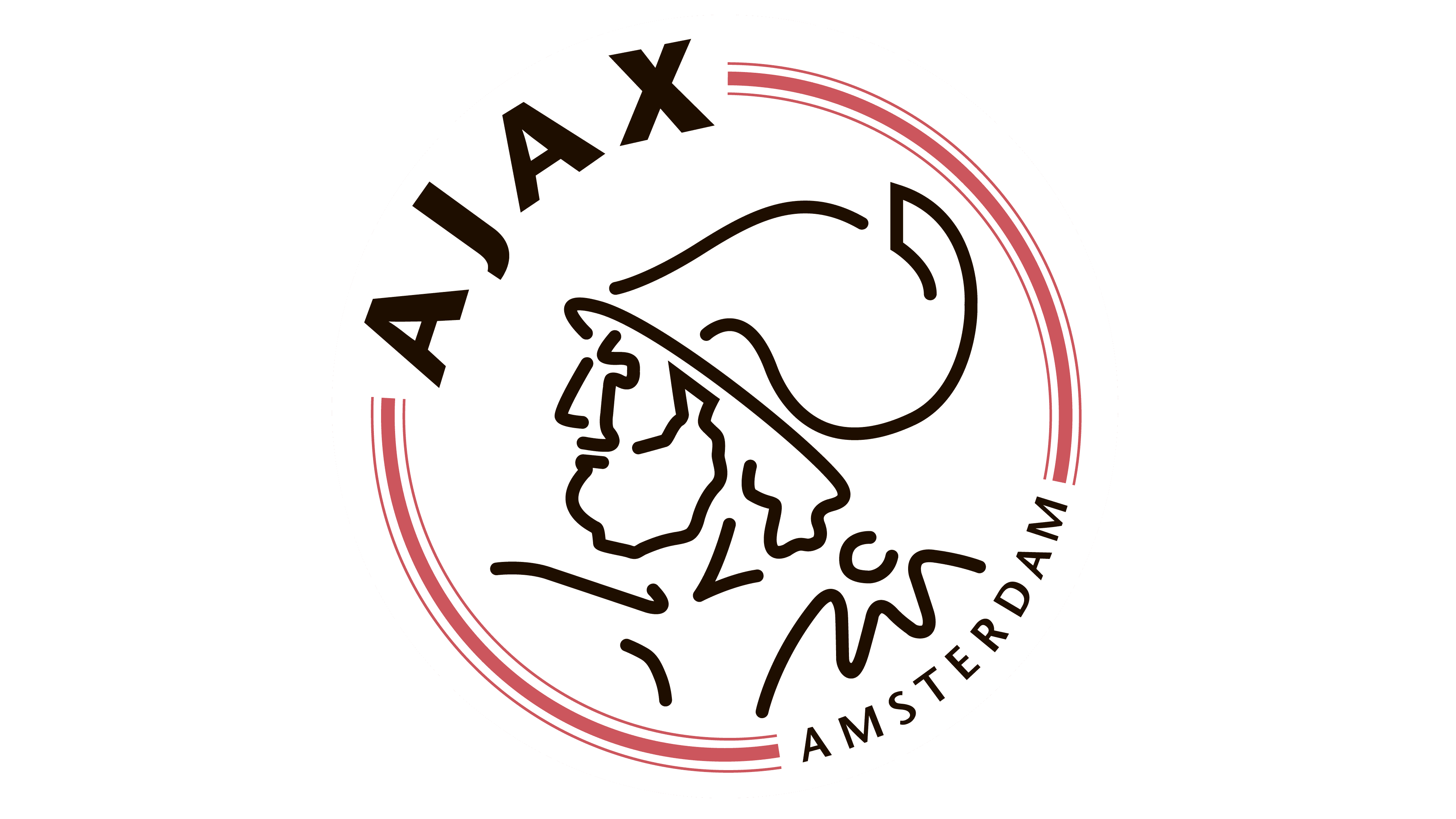
Detail Author:
- Name : Prof. Edward Gislason
- Username : carleton41
- Email : johnnie.kris@medhurst.com
- Birthdate : 1976-11-08
- Address : 12728 Stroman Circles West Shaniamouth, NV 08761
- Phone : 702-916-8302
- Company : Kutch-Durgan
- Job : Technical Director
- Bio : Consequatur magnam nihil eum dolor. Assumenda autem recusandae corporis libero aperiam sed. Laboriosam harum laboriosam aut est dolorem qui alias impedit.
Socials
twitter:
- url : https://twitter.com/barbara.wiegand
- username : barbara.wiegand
- bio : Sunt distinctio voluptatem perspiciatis consequatur. Asperiores sunt magnam nihil illo ipsum corrupti earum. Assumenda cumque a beatae doloremque.
- followers : 4297
- following : 2404
instagram:
- url : https://instagram.com/bwiegand
- username : bwiegand
- bio : Qui quis minus velit omnis non. Sit sunt nam assumenda. Facere impedit cum vel illo itaque at.
- followers : 3916
- following : 2867